After I first thought of making a voltmeter, I needed one thing small, straightforward to hold, and fast to make use of. I imagined it as a software I may hold in my toolbox or use as a part of a fast diagnostic equipment.
The purpose was to create a software that might measure voltages in two ranges (0-3.3V and 0-24V) and connect with Wi-Fi to show the readings in actual time on a webpage.
I needed it to be no greater than the palm of my hand and have the flexibleness to change between the 2 voltage ranges via an online interface.
The answer? IndusVoltmeter – a voltmeter designed across the IndusBoard Coin, which homes the ESP32-S2 chip. The design affords portability, simplicity, and distant connectivity, all wrapped up in a compact bundle.
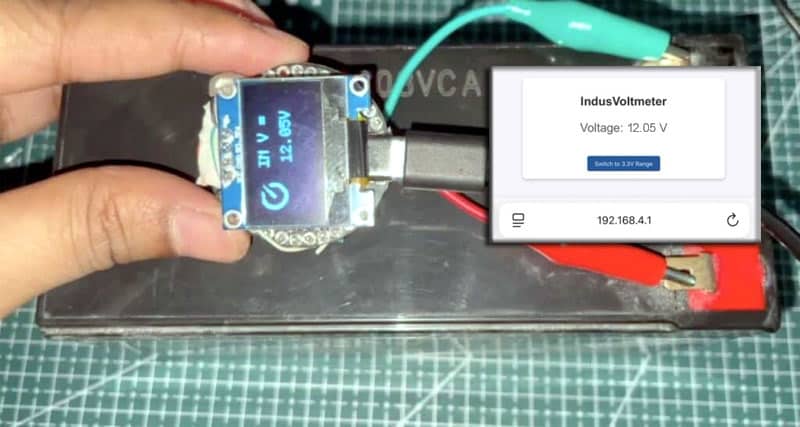
Right here’s how I designed and constructed it.
In immediately’s fast-paced world, having a small, moveable, and handy software is important for fast troubleshooting and initiatives. Whether or not I’m working within the lab, on-site, or at a shopper’s place, the power to hold a voltmeter that doesn’t take up a lot house is a should.
The compact design, about 3 cm in dimension, ensures it’s simply moveable with out compromising performance. And with Wi-Fi connectivity, I can view the measurements remotely, while not having to be bodily near the machine.
Invoice of Materials
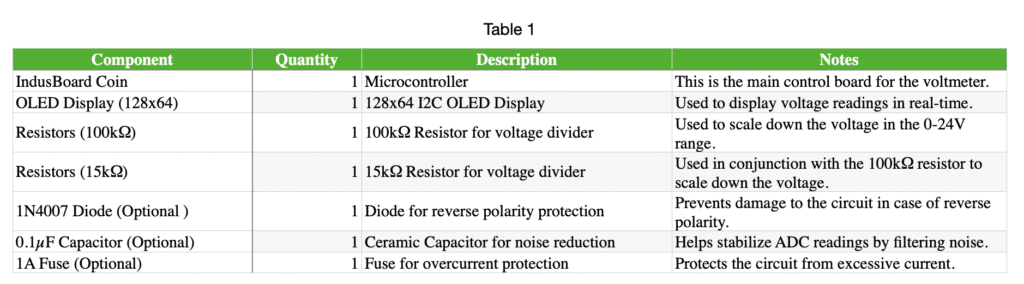
IndusVoltmeter Circuit Design
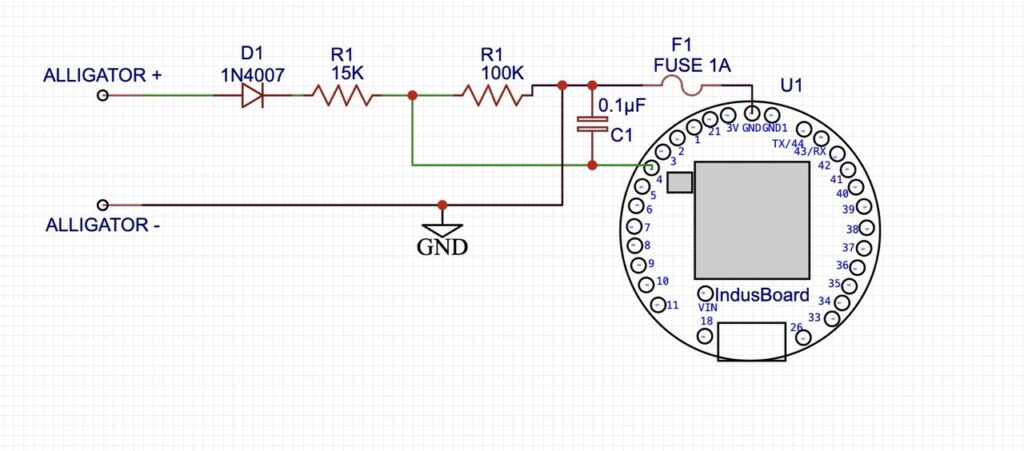
Step 1: Selecting the Voltage Measurement Ranges
The design of the IndusVoltmeter revolves round two voltage ranges:
- 0-3.3V: This vary is for low voltage measurements the place we instantly learn the voltage through the ESP32-S2’s ADC pin. The ESP32 has a 12-bit ADC that may learn voltages on this vary with none modification.
- 0-24V: This vary requires a voltage divider circuit to scale down the voltage. The voltage divider makes use of two resistors to soundly scale excessive voltages (as much as 24V) to the ADC pin, which might solely deal with a most of 3.3V.
Step 2: Creating the Voltage Divider
To measure voltages within the vary of 0-24V, I wanted to make use of a voltage divider to carry down the enter voltage to a stage that the ESP32-S2 may safely learn. The voltage divider consists of two resistors:
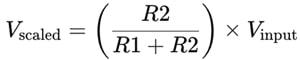

These resistor values scale a 24V enter right down to roughly 3.3V, which is secure for the ESP32’s ADC pin to learn.
Step 3: Including Safety for the Circuit
To make sure that the circuit lasts a very long time, I added safety elements:
- Reverse Polarity Safety: A 1N4007 diode was positioned in sequence with the facility provide. This ensures that the diode will stop injury to the board if the facility is linked incorrectly.
- Overcurrent Safety: I added a 1A fuse to guard towards extreme present, which may in any other case injury elements.
- Noise Discount: To forestall fluctuating readings brought on by electrical noise, I used a 0.1µF capacitor between the ADC pin and GND to stabilize the sign and enhance the accuracy of voltage readings.
Step 4. Including the OLED Show (Non-obligatory)
Since I needed the voltmeter to work with an online interface and in addition present the voltage on a bodily display screen, I made a decision so as to add an OLED show. This show will present the voltage in actual time and assist me shortly verify the readings with out having to entry the net interface.
However you may skip this for those who don’t need it. It nonetheless works and exhibits on the cellphone show when linked to the machine.
I used a 128×64 OLED linked through I2C (SDA, SCL) to the IndusBoard Coin. The show is linked to pins 8 and 9, that are the default I2 pins for the IndusBoard. Test the pinouts of the Indusboard Coin.
Coding IoT-Primarily based Smallest Wi-fi Voltmeter
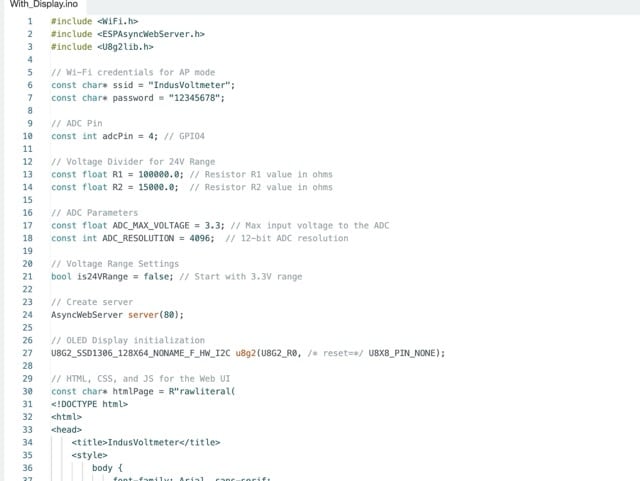
We’ll want to put in a couple of libraries utilizing the Arduino IDE’s library supervisor. First, set up the next libraries:
Now, let’s embody these libraries in our code:
#embody <WiFi.h>
: This library is used to attach the ESP32-S2 to Wi-Fi in AP (Entry Level) mode.#embody <ESPAsyncWebServer.h>
: This library creates an asynchronous net server for serving HTML and dealing with shopper requests.#embody <U8g2lib.h>
: This library is for controlling the OLED show and rendering textual content.
International Variables
- Wi-Fi Credentials: These are the SSID (community identify) and password we’ll use to attach the ESP32-S2 to Wi-Fi in AP mode.
- ADC Pin: The ADC enter pin (GPIO4 on this case) is outlined as `adcPin`.
- Voltage Divider: We’ll use two resistors (R1 and R2) to scale down the voltage from the 24V vary to a secure stage.
- ADC Parameters: We’ll set the utmost ADC voltage (3.3V) and ADC decision (4096, for 12-bit ADC).
- Voltage Vary: We’ll use a boolean flag (`is24VRange`) to point whether or not the voltmeter is within the 24V vary.
Internet Server
- HTML, CSS, and JS for the Internet UI: The HTML construction defines a easy UI with a voltage show and a toggle button to change between the three.3V and 24V ranges. CSS is used to fashion the web page, and JavaScript manages the toggling of the voltage vary and periodically fetches the voltage information from the server.
Setup Perform
- Serial Setup: We’ll initialize the serial communication for debugging functions.
- ADC Configuration: This setting units the ADC decision to 12 bits (4096 ranges) and configures the ADC attenuation to 11 dB. This implies you may learn voltages as much as 3.3V.
- Wi-Fi AP Mode: The ESP32-S2 startsAs an entry level with the SSID “IndusVoThe IP handle of the AP is printed for shopper connection.
- OLED Initialization: Initializes the OLED show to point out voltage readings.
- Internet Server Routes:
- Root Route (“/“): Serves the HTML web page with the voltage show and toggle button.
- Voltage Route (“/voltage”): Reads the analog voltage from the ADC pin, scales it to the suitable vary (both 3.3V or 24V), and sends the end result as JSON.
- Set Vary Route (“/setrange”): Permits the consumer to toggle between the three.3V and 24V voltage ranges. It reads the “range” parameter from the URL and units the is24VRange flag accordingly.
Loop Perform
- Voltage Measurement: The analogRead(adcPin) reads the analog worth from the ADC pin. The uncooked ADC worth is then transformed right into a voltage primarily based on the ADC decision and the vary chosen.
- OLED Show: The measured voltage is proven on the OLED show utilizing the u8g2 library. The voltage is up to date each second.
- Font and Show Setup: Textual content is drawn on the OLED show with a selected font dimension. The voltage worth is up to date in actual time, and the display screen is refreshed each second.
HTML, CSS, and JavaScript
- The webpage shows the present voltage and permits the consumer to toggle between the three.3V and 24V ranges.
- JavaScript fetches the present voltage from the /voltage endpoint each second and updates the show.
- The toggle button sends a request to the /setrange endpoint to vary the voltage vary when clicked, and it updates the button textual content primarily based on the brand new vary.
Testing IndusVoltmeter
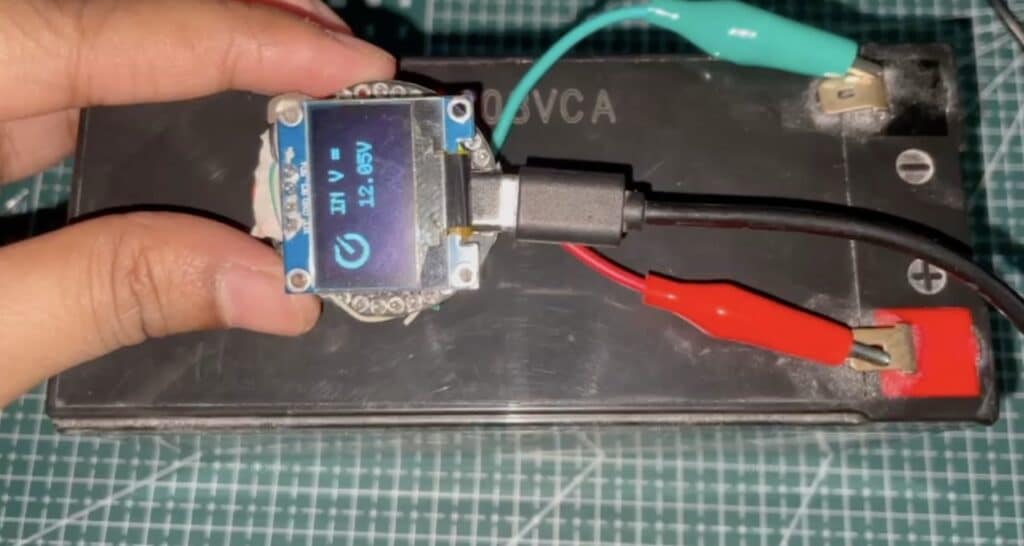
Now, after importing the code, for those who want to use the machine to measure the voltage vary beneath 0 V and three.3 V with out the circuit, you may instantly join the optimistic voltage supply (+) to pin 4 and the detrimental voltage supply (-) to the detrimental I2 GND of the machine.
Please observe that it’s best to cross-check the polarity connections and guarantee they’re appropriate to keep away from quick circuits, as reverse polarity safety just isn’t included on this circuit. You should utilize the circuit diagram so as to add a polarity safety layer and a fuse for short-circuit safety.
If you wish to monitor the voltage repeatedly, you may join the laptop computer to the Indusvoltmeter’s Wi-Fi SSID (password: 1234567890) and seek for the IP handle 192.168.4.1 within the browser. This may show the voltage readings on the laptop computer.
For measuring voltages above 24 V, starting from 0.0 V to 24 V DC, you may create the circuit as described above and join the ADC to the facility supply and floor.
Then, join the machine to the hotspot of the machine and the laptop computer utilizing the password set within the code.
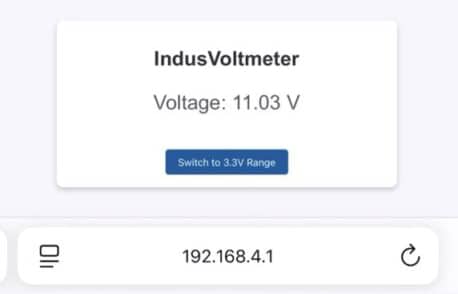
Within the browser, entry the webpage of the machine utilizing the IP handle 192.168.4.1. You possibly can then swap to the 24 V vary utilizing the toggle button on the webpage.