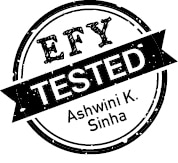
This faculty automation system for reminding the college prayer or nationwide anthem time and period-over bell utilises Arduino to automate the bell schedule and combine the taking part in of the nationwide anthem in faculties or institutes.
The system contains an Arduino microcontroller, an RTC DS3231 module, a microSD card module, and an audio jack to attach the speaker.
POC Video Tutorial In English
POC Video Tutorial In Hindi
Fig. 1 illustrates the core performance of this technique, which eliminates the necessity for guide bell ringing, ensures easy transitions between intervals, and reduces the executive burden on faculty employees. The elements required for setting up this faculty automation system are listed in Desk 1.
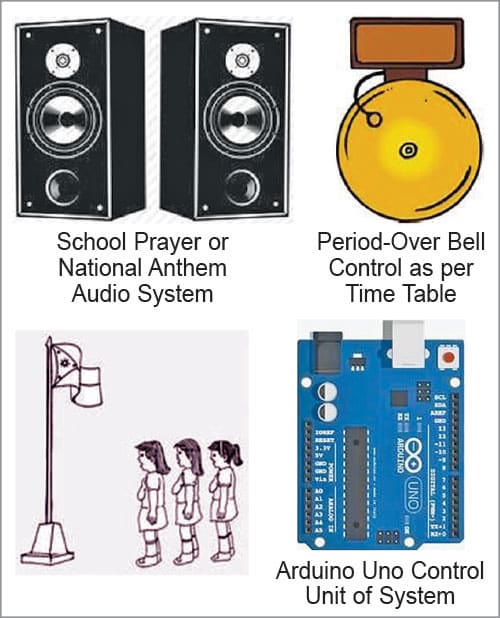
Desk 1: Invoice of Supplies | ||
Elements | Amount | Description |
Arduino Uno | 1 | Management unit of mission |
RTC 3231 | 1 | Actual time clock |
Microcard SD module | 1 | For retailer audio monitor |
5V battery/energy financial institution | 1 | 5V |
3.5mm audio feminine connector | 1 | To interface the speaker |
Software program
The logical software program is written in Arduino IDE. After putting in the associated libraries, together with Wire.h for I2C communication, SD.h for SD card interfacing, and TMRpcm.h for audio playback, add the code.
Earlier than importing the given sketch to the Arduino Uno board, make sure that the right board is chosen. After choosing the port, add the sketch file ‘code.ino’ to the board. Fig. 2 reveals a screenshot of the supply code.
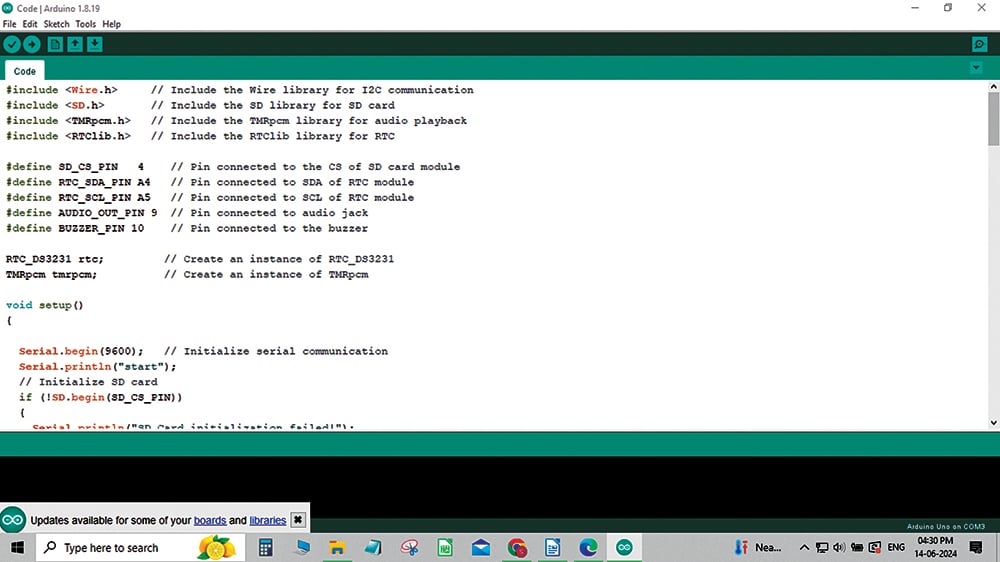
The supplied Arduino program is designed to automate the ringing of a faculty bell and play faculty prayer or nationwide anthem at preset instances. It makes use of numerous libraries and {hardware} elements to attain this performance:
1. RTC (real-time clock): Retains monitor of the present time, even when the Arduino is powered off. It ensures the system operates on the right schedule
2. SD card module: Shops audio information, together with the prayer or nationwide anthem, to be performed by means of a speaker
3. TMRpcm library: Handles audio playback from the SD card
4. Buzzer: Emits a sound at first and finish of every interval to sign the change
Algorithm
1. Initialisation
• Arrange serial communication for debugging
• Initialise the SD card and test for profitable initialisation
• Initialise the RTC and set the present time, if wanted (commented out within the code)
• Arrange the TMRpcm library for audio playback and configure the speaker pin
• Arrange the buzzer pin
2. Principal loop
• Get the present time from the RTC
• Examine the present time towards preset instances for the prayer or nationwide anthem and every interval bell
• If the present time matches a preset time, play the audio or sound the buzzer
• Use a delay to keep away from busy-waiting and scale back CPU utilization
Detailed Code Rationalization
1. Initialisation
Serial.start(9600): Initialises serial communication at a baud fee of 9600 for debugging
SD.start(SD_CS_PIN): Initialises the SD card. If it fails, a message is printed
Wire.start(): Initialises I2C communication for the RTC
rtc.start(): Initialises the RTC. If it fails, a message is printed
tmrpcm.speakerPin = AUDIO_OUT_PIN: Units the pin for audio output
tmrpcm.setVolume(5): Units the amount degree
pinMode(buzzer_pin, output): Units the buzzer pin as an output
2. Principal loop rationalization
rtc.now(): Will get the present time from the RTC
Serial.print(): Prints the present time to the serial monitor for debugging
digitalWrite(buzzer_pin, low): Ensures the buzzer is off initially
Time checks: Checks if the present time matches any of the preset instances. If a match is discovered
playAudio(): Performs the prayer or nationwide anthem, if it’s the beginning time
digitalWrite(buzzer_pin, excessive): Activates the buzzer
delay (30000): Retains the buzzer on for 30 seconds.
digitalWrite(buzzer_pin, low): Turns off the buzzer
delay (1000): Provides a delay of 1 second
Faculty Automation System – Circuit and Working
Fig. 3 reveals the circuit diagram of the automation system. It consists of Arduino Uno, real-time clock, microcard SD module, and three.5mm audio feminine connector. The Arduino program is designed to automate the ringing of bells and taking part in of audio information based on a predefined schedule.
It utilises numerous libraries, together with Wire.h for I2C communication, SD.h for SD card interfacing, TMRpcm.h for audio playback, and RTClib.h for real-time clock performance.
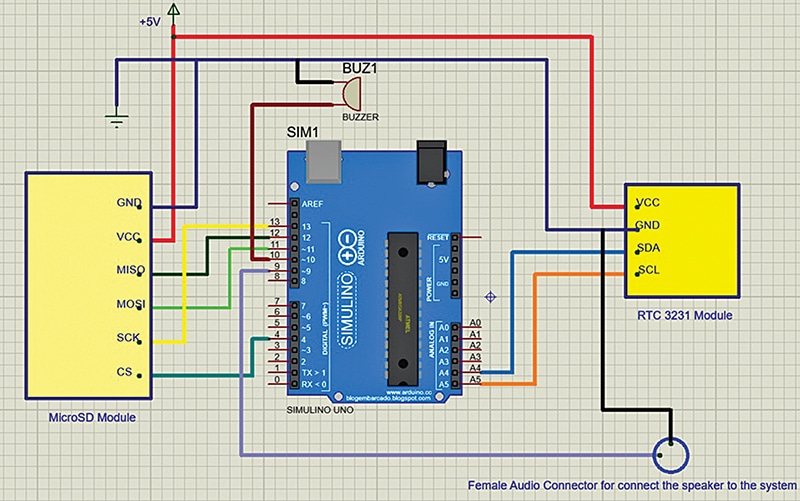
The setup perform initialises serial communication, SD card, RTC module, TMRpcm library, and units the amount degree. It additionally configures the buzzer pin as an output. Within the loop perform, it repeatedly reads the present time from the RTC module and checks if it matches any of the predefined time intervals for bell ringing.
If a match is discovered, it triggers the buzzer, performs the audio file (if obtainable), after which waits for a specified period earlier than turning off the buzzer.
Microcard SD module: The pins on an SD card module serve the next capabilities:
- VCC: This pin offers energy to the SD card module. It’s often related to a 5V or 3.3V energy supply, relying on the module’s specs
- GND: This pin is the bottom connection, offering the reference voltage for the module
- MISO (grasp in slave out): This pin is used for knowledge switch from the SD card to the microcontroller or different system. It carries knowledge from the SD card to the microcontroller when studying knowledge
- MOSI (grasp out slave in): This pin is used for knowledge switch from the microcontroller to the SD card. It carries knowledge from the microcontroller to the SD card when writing knowledge
- SCK (serial clock): This pin is the clock sign generated by the microcontroller for synchronising knowledge switch between the microcontroller and the SD card
- CS (chip choose): This pin is used to allow communication with the SD card. When this pin is about to low (often related to floor), it signifies to the SD card that it ought to hearken to instructions from the microcontroller. The playAudio perform is named to play the audio file saved on the SD card when a bell ringing occasion happens
Actual-time clock (RTC) module: The actual-time clock (RTC) module sometimes consists of a number of pins, every serving a selected perform:
- VCC: This pin is related to the ability provide (often 5V or 3.3V) to supply energy to the RTC module
- GND: This pin is related to the bottom (0V) of the ability provide to finish the circuit
- SDA (serial knowledge): This pin is used for bidirectional serial knowledge switch between the RTC module and the microcontroller. It carries knowledge between the microcontroller and the RTC module throughout communication
- SCL (serial clock): This pin is used to synchronise the info switch between the microcontroller and the RTC module. It generates clock pulses for timing the serial communication
- SQW (sq. wave output) not related: This pin outputs a sq. wave sign with a frequency specified by the RTC module. It may be used as an interrupt sign or for different timing-related functions
- 32kHz output not related: Some RTC modules might have a further pin for outputting a 32kHz clock sign, which can be utilized for timekeeping or different timing-sensitive functions. These pins enable the RTC module to speak with and be managed by the microcontroller, enabling correct timekeeping and different time-related capabilities in embedded techniques
Building and Testing
For the development of the system, first add the supply code `code.ino` into the Arduino Uno board. Then assemble all of the elements based on the circuit diagram as proven in Fig. 3 and Tables 2 and three. The creator’s prototype is proven in Fig. 4.
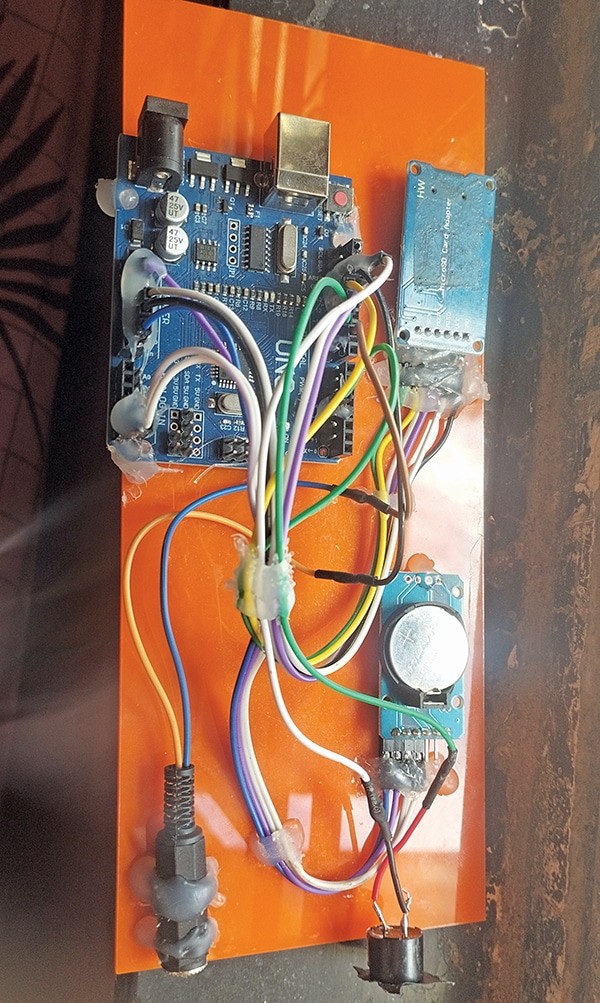
Desk 2: Connecting elements MicroSD | |
Arduino Pins | Pins MicroSD Module and Buzzer |
Arduino pin 4 | CS (chip choose) pin-6 |
Arduino pin 9 | Optimistic pin of audio feminine connector |
Arduino pin 10 | Linked to optimistic pin of buzzer |
Arduino pin 11 | Linked to MOSI pin-4 (grasp out slave in) |
Arduino pin 12 | Linked to MISO pin-3 (grasp in slave out) |
Arduino pin 13 | SCK-pin-5 (serial clock) |
Desk 3: RTC module connection | |
RTC Module | Arduino Pins |
SDA | Linked to Arduino analogue pin-A4 |
SCL | Linked to Arduino analogue pin-A5 |
Vcc | Linked to Arduino analogue pin-A5 |
GND | Linked to Arduino GND pin |
After correct meeting of the system, the decision bell is able to work as follows:
Initially, the circuit is powered up with a 12V exterior energy provide. When faculty beginning time arrives, an announcement for the prayer or nationwide anthem will play.
After that, the bell will ring instantly to point the beginning of faculty.
On the finish of the primary interval (as per the set time in this system), the bell will ring, indicating the top of the primary interval and the beginning of the second interval.
Equally, the designed circuit will monitor the top of every interval and ring the bell accordingly. Repeat from step 1.
As an enchancment, the bell sound will be changed with an audio announcement after every interval, the place the interval quantity is talked about.
Nilesh Ramesh Thakre is Head of the Division at SNJB’s Shri H.H.J.B Polytechnic Chandwad, Dept. of Electronics and Telecommunication Engineering