For those who’re new to Arduino and connecting Arduino to the web, the method might sound sophisticated at first. Many rookies face challenges in relation to web connectivity whereas coding with Arduino. And not using a user-friendly interface and built-in debugging instruments, it may be troublesome to determine and rectify errors. On this article, we are going to present a complete information on connecting your Arduino board to the web, together with the required code.
After I first started enjoying round with my Arduino Uno, I used to be very happy with making LEDs blink and sensors beep. However I quickly realized that the true potential of an Arduino can solely be achieved when you join it to the web.
You possibly can verify the revolutionary Arduino Initiatives.
Just about any intermediate to superior degree Arduino mission would require you to hook up with the web for numerous causes, be it logging information on the cloud reminiscent of climate information, or passing on real-time instructions to your gadget remotely reminiscent of by way of an app in your telephone.
On this tutorial, we’re going to have a look at how one can join your Arduino gadget to the web comprehensively. Every thing—from the {hardware} to the circuit and code—will probably be coated.
Connecting Arduino to the Web with Ethernet
The primary choice for connecting your Arduino to the web is by way of an Ethernet cable. In case you are utilizing an Arduino board that comes with a built-in Ethernet port reminiscent of an Arduino Yún, you possibly can skip the ‘Hardware requirements’ part and the circuit design description given under. Simply join the Ethernet cable to your gadget and begin coding.
Nevertheless, if, like most individuals, you personal a less complicated model of Arduino reminiscent of Arduino Uno that doesn’t have a built-in Ethernet port, you’ll have to purchase a separate gadget, known as an Ethernet defend, to connect to your Arduino.
{Hardware} Necessities
You’ll require an Ethernet cable with the web, an Arduino Uno board, and an
Ethernet Defend. Right here’s how one can join them:
- Join the Ethernet defend on high of the Arduino Uno.
- Join the Ethernet cable to the Ethernet defend.
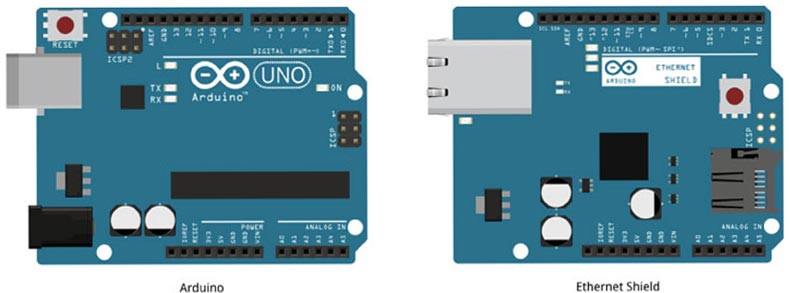
Fig. 1 exhibits Arduino Uno and its Ethernet defend. After connecting the 2, your Arduino Uno ought to appear like the one in Fig. 2.
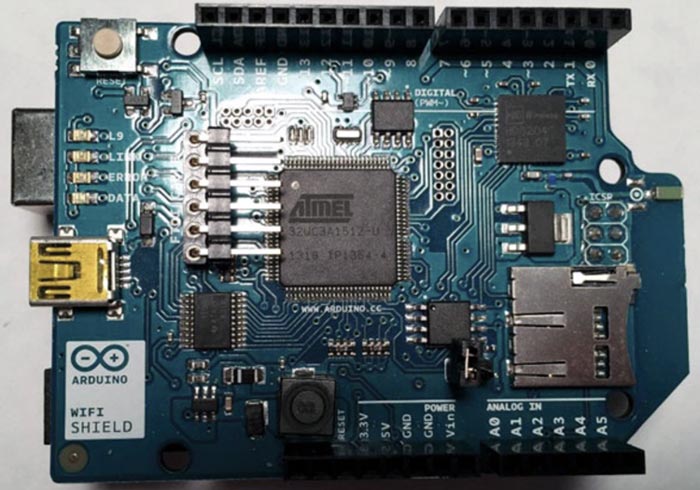
Arduino Code for Connecting to the Web by way of Ethernet
Earlier than getting began with the code for connecting Arduino to the web, we have to embrace an exterior library to be used in this system. This library will assist us set up a connection and ship/obtain information over the web.
Be aware: This library ought to come preinstalled along with your IDE. If for some motive you run into errors, please obtain this library from the official GitHub repository of the Arduino Undertaking.
#embrace <Ethernet.h>
Within the subsequent step, we will probably be defining some constants and variables required for this program.
First, we want the MAC handle. That is typically printed on a sticker on the Ethernet defend. Subsequent, we have to outline a static IP handle.
Ensure that the IP handle that you’re including isn’t being utilized by another consumer. Lastly, we will outline the EthernetClient variable.
byte mac[] = { oxDE, oxAD, oxBE, oxEF,
oxFE, oxED };
IPAddress staticIP(10, 0, 0, 20);
EthernetClient shopper;
We will now write the strategy for connecting to the web. There are two steps right here. First, we attempt to get an IP by way of the DHCP (dynamic host configuration protocol), i.e., attempt to fetch your dynamic IP. If that step fails, then we fall again to the static IP that now we have outlined above.
void connectToInternet()
{
// Step 1 - Strive connecting
with DHCP
If (Ethernet.start(mac) == 0)
{
Serial.print(“[ERROR]
Failed to attach by way of DHCP”);
Ethernet.start(mac,
staticIP); // Join by way of static IP
outlined earlier
}
// Add a delay for initialization
delay(1000);
Serial.println(“[INFO] Connection
Profitable”);
Serial.print(“”);
printConnectionInformation(); // Customized
technique
Serial.print
ln(“---------------------------------”);
Serial.println(“”);
}
As you possibly can see within the code above, we used a customized technique printConnectionInformation() for displaying the connection info. So allow us to go forward and write the code for that.
void printConnectionInformation()
{
// Print IP Tackle
Serial.print(“[INFO] IP Address: ”);
Serial.println(Ethernet.localIP());
// Print Subnet Masks
Serial.print(“[INFO] Subnet Mask: ”);
Serial.println(Ethernet.
subnetMask());
// Print Gateway
Serial.print(“[INFO] Gateway: ”);
Serial.println(Ethernet.gatewayIP());
// Print DNS
Serial.print(“[INFO] DNS: ”);
Serial.println(Ethernet.
dnsServerIP());
}
Lastly, we will write the usual features for this system, i.e. setup() and loop().
void setup()
{
Serial.start(9600);
// Hook up with the web
connectToInternet();
}
void loop()
{
// Nothing a lot to do right here.
}
If all the things checks out, try to be seeing an output much like Fig. 3 on the serial monitor window.
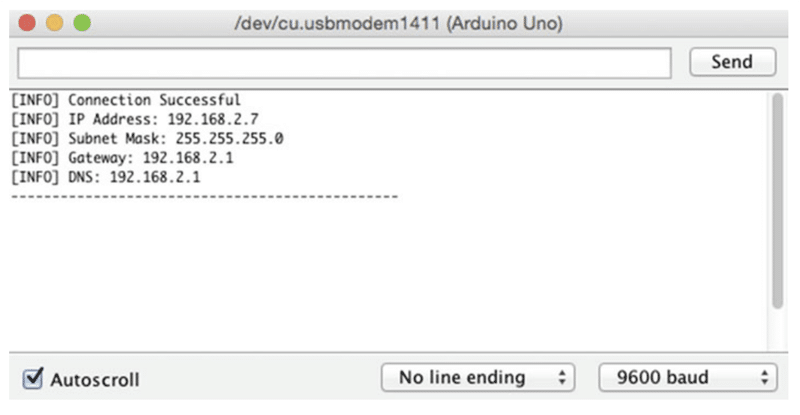
Connecting Arduino to the Web by way of Wi-Fi
The second choice is to attach your Arduino to the web wirelessly by way of Wi-Fi. If, like most individuals, you personal an Arduino Uno or another Arduino board that doesn’t have a built-in Wi-Fi functionality, you’ll have to purchase a wi-fi defend individually, just like the Ethernet defend.
For those who personal an Arduino Yún or another Arduino board with built-in wi-fi functionality, you possibly can skip the ‘Hardware requirements’ and the outline on the subsequent web page and get began with the code immediately.
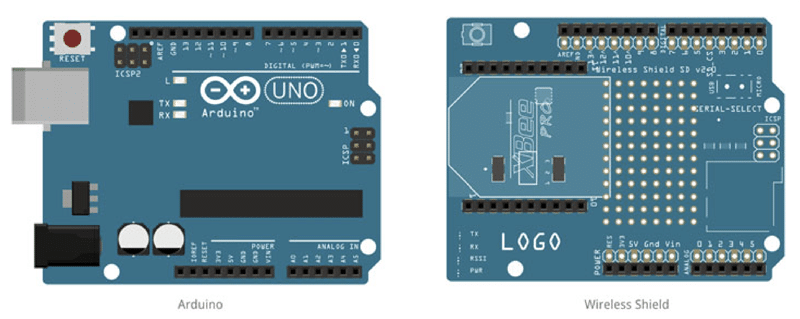
{Hardware} Necessities
You will have an Arduino Uno and a wi-fi defend. Right here’s how one can join them:
- Join the wi-fi defend on high of the Arduino Uno.
- Join the Arduino Uno to your laptop by way of the USB port.
Fig. 4 exhibits the Arduino Uno and the wi-fi defend. If all the things is linked correctly, your Arduino Uno board ought to appear like the one in Fig. 5.
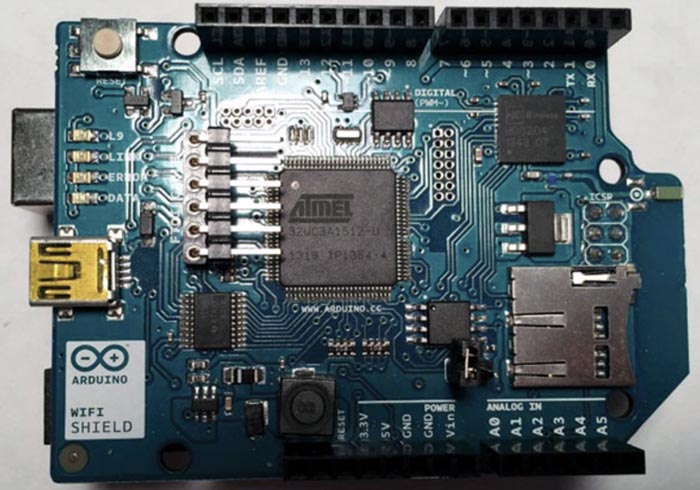
Arduino Code for Connecting to the Web by way of Wi-Fi
When connecting to the web by way of Ethernet, we used the exterior library. Equally, for connecting to the web wirelessly, we will be utilizing the exterior library.
Be aware: This library ought to come preinstalled along with your IDE. If for some motive you run into errors, please obtain this library from the official GitHub repository of the Arduino Undertaking.
#embrace <SPI.h>
#embrace <WiFi.h>
Subsequent, we will outline the constants and variables required for connecting wirelessly. For connecting with the Wi-Fi, we require the ssid and password of the Wi-Fi that we will be utilizing. We will additionally create a WiFiClient variable for connecting to the web.
char ssid[] = “Write WiFi SSID here”;
char cross[] = “Write WiFi password
right here”;
int keyIndex - 0;
int standing = WL_IDLE_STATUS;
WiFiClient shopper;
Now we will outline some customized strategies for connecting and sending/receiving information over the Web. First, allow us to create the strategy connectToInternet() for connecting with the Web.
void connectToInternet()
{
standing = WiFi.standing();
if (standing == WL_NO_SHIELD)
{
Serial.println(“[ERROR] WiFi Defend
Not Current”);
whereas (true);
}
whereas ( standing != WL_CONNECTED)
{
Serial.print(“[INFO] Trying
Connection - WPA SSID: ”);
Serial.println(ssid);
standing = WiFi.start(ssid, cross);
}
Serial.print(“[INFO] Connection
Profitable”);
Serial.print(“”);
printConnectionInformation();
Serial.print
ln(“-------------------------”);
Serial.println(“”);
}
As you possibly can see within the code above, now we have known as the customized technique printConnectionInformation() to show the details about our Wi-Fi connection. So allow us to go forward and write that technique:
void printConnectionInformation()
{
Serial.print(“[INFO] SSID: ”);
Serial.println(WiFi.SSID());
// Print Router’s MAC handle
byte bssid[6];
WiFi.BSSID(bssid);
Serial.print(“[INFO] BSSID: ”);
Serial.print(bssid[5], HEX);
Serial.print(“:”);
Serial.print(bssid[4], HEX);
Serial.print(“:”);
Serial.print(bssid[3], HEX);
Serial.print(“:”);
Serial.print(bssid[2], HEX);
Serial.print(“:”);
Serial.print(bssid[1], HEX);
Serial.print(“:”);
Serial.println(bssid[0], HEX);
// Print Sign Energy
lengthy rssi = WiFi.RSSI();
Serial.print(“[INFO] Sign Energy
(RSSI) : ”);
Serial.println(rssi);
// Print Encryption kind
byte encryption = WiFi.encryption
Kind();
Serial.print(“[INFO] Encryption Kind
: ”);
Serial.println(encryption, HEX);
// Print WiFi Defend’s IP handle
IPAddress ip = WiFi.localIP();
Serial.print(“[INFO] IP Address : ”);
Serial.println(ip);
// Print MAC handle
byte mac[6];
WiFi.macAddress(mac);
Serial.print(“[INFO] MAC Address: ”);
Serial.print(mac[5], HEX);
Serial.print(“:”);
Serial.print(mac[4], HEX);
Serial.print(“:”);
Serial.print(mac[3], HEX);
Serial.print(“:”);
Serial.print(mac[2], HEX);
Serial.print(“:”);
Serial.print(mac[1], HEX);
Serial.print(“:”);
Serial.println(mac[0], HEX);
}
Lastly, allow us to write down the usual features, i.e. setup() and loop():
void setup()
{
Serial.start(9600);
// Hook up with the web
connectToInternet();
}
void loop()
{
// Nothing to do right here
}
Hurray! We’re executed with the Arduino code for connecting to the web by way of Wi-Fi. In case there are not any errors, the output in your serial monitor window ought to appear like the output in Fig. 6.
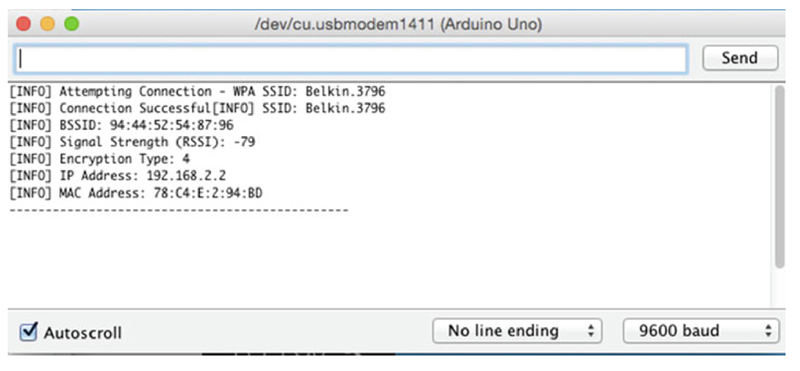
This text was first printed within the December 2022 concern of Open Supply For You journal.
Mir H.S. Quadri is a analysis analyst with a specialization in synthetic intelligence and machine studying. He’s the founding father of Arkinfo, which focuses on the analysis and growth of tech merchandise utilizing new-age applied sciences. He shares a deep love for evaluation of technological tendencies and understanding their implications. Being a FOSS fanatic, he has contributed to a number of open-source initiatives